mirror of
https://codeberg.org/forgejo/forgejo.git
synced 2025-04-25 04:57:31 +00:00
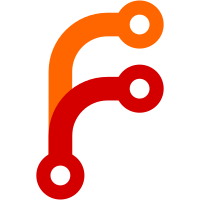
## Checklist The [contributor guide](https://forgejo.org/docs/next/contributor/) contains information that will be helpful to first time contributors. There also are a few [conditions for merging Pull Requests in Forgejo repositories](https://codeberg.org/forgejo/governance/src/branch/main/PullRequestsAgreement.md). You are also welcome to join the [Forgejo development chatroom](https://matrix.to/#/#forgejo-development:matrix.org). ### Tests - I added test coverage for Go changes... - [x] in their respective `*_test.go` for unit tests. - [ ] in the `tests/integration` directory if it involves interactions with a live Forgejo server. ### Documentation - [ ] I created a pull request [to the documentation](https://codeberg.org/forgejo/docs) to explain to Forgejo users how to use this change. - [ ] I did not document these changes and I do not expect someone else to do it. ### Release notes - [ ] I do not want this change to show in the release notes. - [ ] I want the title to show in the release notes with a link to this pull request. - [ ] I want the content of the `release-notes/<pull request number>.md` to be be used for the release notes instead of the title. Co-authored-by: Michael Jerger <michael.jerger@meissa-gmbh.de> Reviewed-on: https://codeberg.org/forgejo/forgejo/pulls/7203 Reviewed-by: Earl Warren <earl-warren@noreply.codeberg.org> Co-authored-by: zam <mirco.zachmann@meissa.de> Co-committed-by: zam <mirco.zachmann@meissa.de>
90 lines
2 KiB
Go
90 lines
2 KiB
Go
// Copyright 2024 The Forgejo Authors. All rights reserved.
|
|
// SPDX-License-Identifier: MIT
|
|
|
|
package forgefed
|
|
|
|
import (
|
|
"strings"
|
|
"testing"
|
|
"time"
|
|
|
|
"forgejo.org/modules/validation"
|
|
)
|
|
|
|
func Test_FederationHostValidation(t *testing.T) {
|
|
sut := FederationHost{
|
|
HostFqdn: "host.do.main",
|
|
NodeInfo: NodeInfo{
|
|
SoftwareName: "forgejo",
|
|
},
|
|
LatestActivity: time.Now(),
|
|
HostPort: 443,
|
|
HostSchema: "https",
|
|
}
|
|
if res, err := validation.IsValid(sut); !res {
|
|
t.Errorf("sut should be valid but was %q", err)
|
|
}
|
|
|
|
sut = FederationHost{
|
|
HostFqdn: "",
|
|
NodeInfo: NodeInfo{
|
|
SoftwareName: "forgejo",
|
|
},
|
|
LatestActivity: time.Now(),
|
|
HostPort: 443,
|
|
HostSchema: "https",
|
|
}
|
|
if res, _ := validation.IsValid(sut); res {
|
|
t.Errorf("sut should be invalid: HostFqdn empty")
|
|
}
|
|
|
|
sut = FederationHost{
|
|
HostFqdn: strings.Repeat("fill", 64),
|
|
NodeInfo: NodeInfo{
|
|
SoftwareName: "forgejo",
|
|
},
|
|
LatestActivity: time.Now(),
|
|
HostPort: 443,
|
|
HostSchema: "https",
|
|
}
|
|
if res, _ := validation.IsValid(sut); res {
|
|
t.Errorf("sut should be invalid: HostFqdn too long (len=256)")
|
|
}
|
|
|
|
sut = FederationHost{
|
|
HostFqdn: "host.do.main",
|
|
NodeInfo: NodeInfo{},
|
|
LatestActivity: time.Now(),
|
|
HostPort: 443,
|
|
HostSchema: "https",
|
|
}
|
|
if res, _ := validation.IsValid(sut); res {
|
|
t.Errorf("sut should be invalid: NodeInfo invalid")
|
|
}
|
|
|
|
sut = FederationHost{
|
|
HostFqdn: "host.do.main",
|
|
NodeInfo: NodeInfo{
|
|
SoftwareName: "forgejo",
|
|
},
|
|
LatestActivity: time.Now().Add(1 * time.Hour),
|
|
HostPort: 443,
|
|
HostSchema: "https",
|
|
}
|
|
if res, _ := validation.IsValid(sut); res {
|
|
t.Errorf("sut should be invalid: Future timestamp")
|
|
}
|
|
|
|
sut = FederationHost{
|
|
HostFqdn: "hOst.do.main",
|
|
NodeInfo: NodeInfo{
|
|
SoftwareName: "forgejo",
|
|
},
|
|
LatestActivity: time.Now(),
|
|
HostPort: 443,
|
|
HostSchema: "https",
|
|
}
|
|
if res, _ := validation.IsValid(sut); res {
|
|
t.Errorf("sut should be invalid: HostFqdn lower case")
|
|
}
|
|
}
|